|

|
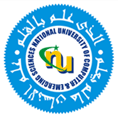
|
Course: Design & Analysis of Algorithms
Type: Computer Science Core
Credit Hours: 4
Semester: Spring 2003
|
Course Objectives

-
To develop an understanding of the concepts and complexities of algorithms,
so the
students can appreciate the requirement of fast and efficient algorithms.
Cover different
sorting and graph algorithms, along with the concept of theory of NP
completeness, so
that students can identify computationally intractable problems.
-
The main focus will be looking at the algorithms from an applied
perspective, this
includes coding algorithms using efficient data structures, running
simulations and
comparing results.
-
Tasks/capabilities the students should be able to undertake upon completion
of
this course are:
-
prepare the students for the market as better and smart programmers who are
able to differentiate between the tasks that can be solved or not
-
enable the students to comprehend problems where ever the quality of a
solution has to be judged
-
prepare the students to undertake graduate level course work where they
may have to take another advance course in algorithms
Course Outline

- Overview
- Introduction to Algorithms
- Complexity Analysis
- Program Efficiency and Complexity
- Theory and Analysis of Recursive Algorithms
- Graph Theory
- Sorting Algorithms
- Quick Sort
- Heap Sort
- External Merge Sort
- Linear Time Sorting
- Data Structures
- Elementary Data Structures
- Hash Tables
- Binary Search Tree
- B-Trees
- Advanced Design and Analysis Techniques
- Data Mining
- Divide and Conquer Algorithms
- Greedy Algorithms
- Dynamic Programming
- Graph Algorithms
- Elementary Graph Algorithms
- Graph Drawing
- Minimum Spanning Trees
- Single Source and all pair Shortest Paths
- NP Completeness
- Efficient Reductions
- Theory of NP Completeness
Text Books

-
Cormen, Leiserson and Rivest, "Introduction to Algorithms", MIT Press, 1990
-
Aho, Hopcroft and Ullman, "Data Structures and Algorithms", Addison-Wesley, 1983
|