TABLE OF
CONTENT
- Client Server Architecture
- CLI, ODBC, JDBC
- JDBC Core Classes
- JDBC Security
- JDBC Architecture
- JDBC Drivers
- A Breif description of JDBC Classes
- JDBC 2-Tier Architecture
- JDBC 2-Tier Architecture with CORBA
- DbANYWHERE 3-Tier Architecture
- JDBC-CORBA 3 -Tier Architecture
- JDBC-CORBA 2-Tier Case Study
- Summary(Comparasion 2-Tier and 3-Tier)
Appendix A
(Installing and running the Debit Credit 2-Tier Application)
Appendix B
(References)
Appendix C
(Source Code)
1.0 Client/Server Software Architecture
It is becoming increasingly rare to carry out any given computing task using a single
computer in isolation. Most often, whether or not the user is aware of it, part of the
task performed takes place on one or more other computers networked to the user's
computer.
As network computing has developed, it has become more common for different types of
computers to specialize in specific areas. Some computers do nothing but store archives of
files and software for on-demand use by network users, some computers specialize in
super-high-resolution graphics for desktop design and engineering, others focus on
high-volume centralized database transaction processing, and many simply function as
general purpose workstations which present graphical user interfaces to all these services
to end-users.
This new diversification of network computing tasks has resulted in a new general
paradigm for software architecture called client/server. In this architecture, most
software applications have exactly two components: the Client component and the Server
component.
Client
A client is a member of a class of programs in a modern software architecture
paradigm called client/server software Architecture in which all applications have two
components: the client component and the server component. The client component
typically has the following characteristics:
- A client is executed on-demand; only when there is a need for it (contrasted with a
server which usually is always executing).
- A client is active; it initiates all dialogue to a server as needed to perform its
tasks. (A server is passive and waits for requests, never initiating any communications
with a client).
- A client interfaces with humans (whereas a server normally runs in the background and
interfaces only with other software (clients)).
- At any given time there probably numerous instances of the client component of the
application running on different computers throughout the network, all of which are
connecting to only one or two servers (a server typically is a centralized process which
serves many clients).
Server
A server is a member of a class of programs in a modern software architecture
paradigm called client/server software Architecture, in which all applications have two
components: the client component and the server component. The server component
typically has the following characteristics:
- A server is most often centralized, usually on a large computer (a client usually runs
in multiple instances on desktops or other small stations throughout a network.)
- A server is passive; it does little or nothing until it receives an explicit request
from a client to do something. (A client is active since it's a tool in the hands of a
human).
- A server usually runs continuously waiting for requests, while a client runs only
on-demand (when invoked by a human).
- A server usually runs in the background and interfaces only with other software
(clients), and rarely interfaces directly with humans (whereas a client is usually invoked
by a human and takes commands from a human).
- A server must handle multiple tasks concurrently since many clients may be requesting
it's services simultaneously. A client usually works for one person and does one thing
after another in sequence, as the user commands it.
Client Server Architecture
- In this 3 -tier connection the Front end can be written in any Language(Java, C++,
SmallTalk).
- Middle tier where most of your business logic resides, can be any object(Java, C++,
SmallTalk).
- The connectivity between the first tier and the second tier uses different connection
protocol (COM, CORBA, RMI, HTTP).
- The connectivity to the second and third tier can be used by ODBC or JDBC or JDBC-ODBC
bridge. Here you can all used different connection protocol (COM ,CORBA etc ) which leads
to the four tier Architecture.
2.0 CLI(Called Level Interface)
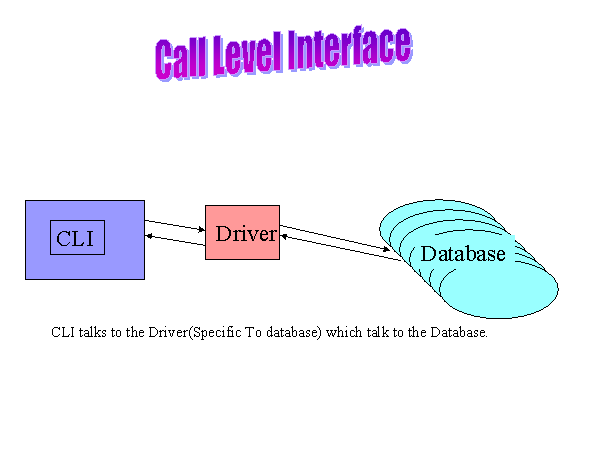
The CLI requires the use of intelligent database drivers that accept a CLI call and
translate it into the native database Server's access language. With the proper driver any
Front end can access the Database with the help of Called Level Interface. CLI is a
procedure interface to the SQL. You write SQL to query over the database. So CLI is a SQL
Wrapper. CLI provides a driver Manager that talks to a driver through a Service Provider
Interface(SPI).
OBDC (Open DataBase Connectivity )
ODBC is also based on the SQL CLI .
Abbreviation of Open DataBase Connectivity, a
standard database access method developed by Microsoft . The goal of ODBC is to make it
possible to access any data from any application , regardless of which database management
system is handling the data. ODBC manages this by inserting a middle layer, called a
database driver, between an application and the DBMS. The purpose of this layer is
to translate the application's data queries into commands that the DBMS understands. For
this to work, both the application and the DBMS must be ODBC-compliant -- that is,
the application must be capable of issuing ODBC commands and the DBMS must be capable of
responding to them.
JDBC(Java DataBase Connectivity)
JDBC is based on the SQL CLI which is also the origin of Microsoft ODBC.
JDBC is simply a JAVA object interface to SQL.
JDBC add a Java SQL Wrapper to the database.
JDBC is portable SQL CLI written entirely in Java.
JDBC uses a driver Manager to automatically load the right driver to talk to a given
database.
3.0 JDBC Core Classes
JDBC specification includes eight new Java interfaces and ten new classes
These are divided into following four groups
- JDBC Core interfaces and classes
- Java Language Extension
- Java Utility Extension
- SQL Metadata Interface
JDBC Core Interfaces and classes
These are seven Interfaces and two Classes. These are the interfaces and abstract
classes all JDBC drivers must implements. You used these classes to locates local or
remote database, established the connection, submit queries, process the result set.
Driver Interface: This class is used for locating the driver for the database.
Driver Manager Class: This class is used at run time to load the driver objects and
creates new database connection Object.
DriverPropertyInfo Class: This is the special class to get the meta information
about the driver.
Connection Interface: You use the connection object to connect to the database. You
can connect to the multiple database or single database. Connection class is used for the
commit and rollback.
Statement Interface: Statement objects are used to execute SQL on a given
Connection object.
Prepared Statement Interface : Prepared Statement Interface object is precompiled
SQL and is used to execute the same SQL statement multiple times using different
parameter. There are series of method to set the parameter fields of the Prepared
Statement (setXXX).
Callable Statement Interface: These objects are used for the stored procedure.
Result Set Interface: Result set object encapsulates the rows returned by the
database when you execute the query on the Statement Object. The object maintains a cursor
that points to the current row of data. We can move this cursor forward and backward. You
can retrieved the data from the result set into Java object by using getXXX.
Result Set Meta Data: You used this object to retrieve number, types, properties of
the Result Set.
JDBC Language Extension
These contains four classes.
Bignum Class: This class is used to stores a high precision of data hence JDBC has
a new class with the floating point capabilities.
The SQLException class, SQLWarning class and DataTruncation class
are used for Exception handling in the JDBC.
JDBC Java Utilities
This contains three classes. As database used fine-grained time and date utilities so
JDBC has a new classes which are extension of Date class of java.util.
It has Date, Time, TimeStamp classes.
JDBC Metadata
All the commercial database provides information at the run time ,So to discover the
information of the database at run time you need DataBaseMeta Class.
4.0 JDBC Security
JDBC driver is prevented an untrusted applet from accessing databases outside the home
machine.
Java Application can load the driver from the local class path and allows the
application free access the remote server.
JDBC driver Check the Shared TCP Connection. If you JDBC driver loaded from the applet
try to open an TCP/IP connection on the set of machine that an applet is allowed to call.
Worse case using Native methods. In case of ODBC-JDBC bridge might use low level
database libraries has a difficult to determine what files or network connection will be
opened by the low level libraries. In such case the driver must make a worst case security
assumptions and deny all database access to downloads applet unless the driver is
completely confident that intended access is not fatal.
5.0 JDBC Architecture
- JDBC is a portable SQL CLI Written entirely in Java. It Lets you write database
independent Java code.
- It has different layered architecture which helps user to communicate with the database.
- Java Application /Applet talks to the JDBC API which talks to the Driver Manager, so
Driver Manager is the layers which connects user to the database.
- As we see that the Server Provider API and different Drivers are implemented at the
lower level which helps in loading right Driver and talk to the database.
6.0 JDBC Drivers
There are basically four types of JDBC Drivers
- The JDBC-ODBC bridge provides JDBC access via most ODBC drivers.
- A native-API partly-Java driver
- A net-protocol all-Java driver
- A native-protocol all-Java driver
S.No. |
Driver Category |
ALL JAVA ? |
NET PROTOCOL |
1 |
JDBC-ODBC Bridge |
No |
Direct |
2. |
Native API |
No |
Direct |
3. |
JDBC- Net |
Yes |
Requires Connector |
4 |
Native protocol as basis |
Yes |
Direct |
- JDBC -ODBC Bridge Driver This types of driver used JDBC to talk to ODBC Driver. Note
that some ODBC binary code and in many cases database client code must be loaded on each
client machine that uses this driver.
- A native-API partly-Java driver converts JDBC calls into calls on the client API
for Oracle, Sybase, Informix, DB2, or other DBMS. Note that, like the bridge driver, this
style of driver requires that some binary code be loaded on each client machine.
- A net-protocol all-Java driver translates JDBC calls into a DBMS-independent net
protocol which is then translated to a DBMS protocol by a server. This net server
middleware is able to connect its all Java clients to many different databases.
- A native-protocol all-Java driver converts JDBC calls into the network protocol
used by DBMS directly. This allows a direct call from the client machine to the DBMS
server and is a practical solution for Intranet access.
7.0 A Brief Description of JDBC Core Classes
JDBC URLs
jdbc:<subprotocol>:<subname>
The three parts of a JDBC URL are broken down as follows:
jdbc - The protocol in a JDBC URL is always jdbc.
<subprotocol>- The name of the driver or the name of a database connectivity
mechanism, which may be supported by one or more drivers. A prominent example of a
subprotocol name is "ODBC", jdbc:odbc:oracle.
<subname>-a way to identify the database. The subname can vary, depending on
the subprotocol, and it can have a subname with any internal syntax the driver writer
chooses. The point of a subname is to give enough information to locate the database.
Address should be included in the JDBC URL as part of the subname and should follow the
standard URL naming convention of //hostname:port/subname. Supposing that "msql"
is a protocol for connecting to a host on the Internet, a JDBC URL might look like this: jdbc:msql://doc.acadiau.ca :8099/Mydatabase.
Connection Object
A connection to a database is made with a call to the method
DriverManager.getConnection(). This method uses a string containing an URL.
The DriverManager class, referred to as the JDBC management layer, attempts to locate a
driver than can connect to the database represented by that URL.
String url = "jdbc:odbc:oracle7";
Connection con =
DriverManager.getConnection(url,"userid","passwd");
Driver Manager
Driver Manager class is the management layer between User and Driver.
Driver manager class implements Single tone Design Pattern.
All methods are Static.
To load a driver and register (Explicitly). Class.forName("jdbc.odbc.JdbcOdbcDriver");
Java keep tracks of all the drivers Registered(java.lang.System properties jdbc:drivers = foo.bah.Driver:wombot.sql.Driver ).
In order to connect to the database Driver manager makes calls to all of the registered
drivers. It calls the connect method of each driver internally which returns the
connection object if connected successfully.
Driver
It is implemented with the special static section which creates an instance of it and
calling DriverManager.registerDriver(MyDriver()).
Static {
java.sql.DriverManager.registerDriver(new MyDriver());
.
}
Most of time you do not deal with the Driver.
Driver manager uses getConnection() method which uses the connect() method of all the
registered drivers to find the database for a given URL and return the connection object
if connected.
Statement
Statement- -created by the method createStatement. A Statement object is used for
sending simple SQL statements.
createStatement method is used for simple SQL statements (no parameters).
Connection con =
DriverManager.getConnection(url,"userid","password");
Statement stmt=con.createStatement();
ResultSet rs = stmt.executeQuery("Select * from MyDatabasetable");
PreparedStatement- -created by the method prepareStatement. A PreparedStatement object
is used for SQL statements that take one or more parameters as input arguments.
PrepareStatement method is used for SQL statements with one or more IN parameters and
are used for SQL statements that are executed frequently.
CallableStatement- -created by the method prepareCall. CallableStatement objects are
used to execute SQL stored procedures- -a group of SQL statements that is called by name,
much like invoking a function at the database. It can have both IN and OUT parameter.
prepareCall method is used for calling the stored procedures at the database end.
There are three different methods used for the Statement Object
execute return More than one result set
executeUpdate used for table update SQL,DLL(CREATE TABLE,DROP TABLE)etc
executeQuery Single Result Set
Result Set
Result set returns all the rows which specified the conditions set in the statement
object.
Resultset maintains the cursor which points to the current row.
Resultset next() method will move the cursor to the next row..
DataBaseMetaData
It provides information of database as a whole.
Once you create an instance of it,You can call different method to retrieve information
about database .
DatabaseMetaData dbmd = con.getMetaData();
It has aroud 133 Methods which tells about the database you have connected.
ResultSetMetaData
It tell the information about result set such column properties, table name, no of rows
effective etc.
ResultsetMetaData rsmd=rs.getMetaData();
SQL Mapping
As JDBC is wrapper class for Database object so there are two types of Mapping in order
to have compatibility between Java Objects and Database.
1. Java to SQL(e.g.: String to VARCHAR or CHAR)
2. SQL to Java(e.g.: SMALLINT to Integer)
JDBC 2 Tier Architecture

- 2-Tier is based on the Fat Client Approach, the client maintains the JDBC drivers for
every database engine it needs.
- In a pure 2 -Tier approach the application logic runs on the client. In less pure 2
-Tier approach you can put some logic on the database in form of stored procedures(which
JDBC supports).
- It has some drawbacks such as it is slow, difficult to scalable, security is low etc.
9.0 JDBC -CORBA 2-Tier Plus Architecture
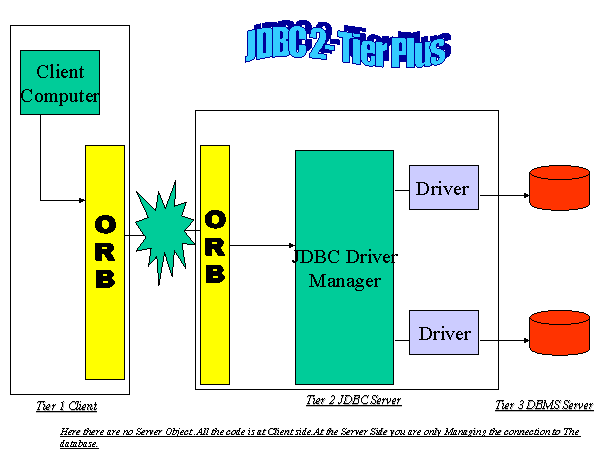
- In this case at the Server Side we are managing the Driver Manager and the Driver. There
are no Server Object in this case.
- Client invokes normal JDBC calls which is transferred to the JDBC driver Server(middle
tier) via ORB or RPC to the JDBC Driver Manager on the Server side.
- The example of RPC is Symantec Café which uses sockets based RPC to communicate between
the client and the Server
10.0 DbANYWHERE 3-tier Architecture

- dbANYWHERE middleware software supports a 3-tier architecture for the database access.
- Each layered architecture refers to the database client(Application or Applet),
dbANYWHERE server, and database Server. You can run all of them on One Machine.
- Using of dbANYWHERE we can have our first tier database independent. All the calls from
the clients are handles by the dbANYWHERE which in turns passes it to the database.
- All the three tiers uses network Configuration to talk to each other.
- In general it talk at Port no 8889 at your IP address
11.0 JDBC- CORBA 3-tier Architecture
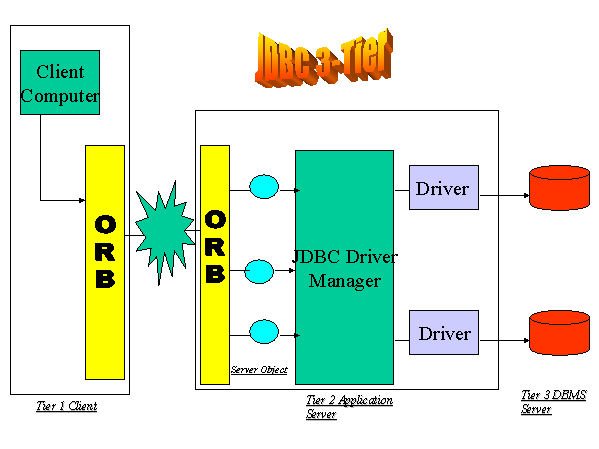
- In this type of Implementation the Client talks to the Server Object using ORB. Client
has nothing to deals with the database. Any function that deals with the database is on
the server Side.
- On the Server Side, the Server Object gets the request from the client and Load the
Appropriate Driver for the database and with the help of JDBC Driver Manager and get
connected to the Database.
12.0 JDBC CORBA 2 -Tier CASE Study
Application
Debit and Credit
Approach
In this application we have a database called Bank. We use CORBA to organize the
Benchmark. Client is updating the database using the JDBC API. Clients are also
registering there callbacks to the Server Coordinator via CORBA. Finally the Coordinator
uses CORBA to controls the Clients. MultiConsole (Applet) controls the Coordinator via
CORBA. It is totally multithreaded scenario in a CORBA environment.
Players at Server Side
MultiConsole is the client applets that also serves as the control panel for
Coordinator(Server). You can use this applets to tells the clients when to stop and start
invoking the methods .
Coordinator is the Server application that knows how to runs the Clients. It tells
ours clients when to start and stop. The clients must registered with the Server
(Callbacks) inorder to received method invocations by the server. Coordinator tells each
clients to start and start the JDBC invocations. MultiConsole is the Control panel for the
server Coordinator
The IDL interface for the Coordinator is
module MultiCoordinator
{ interface Coordinator
{ // object for registering and controlling client counting
boolean register(in string clientNumber,in Client::ClientControl clientObjRef);
boolean start();
string stop();
};
};
Here we are using the register( ) method to register the client on the Coordinator
Server for the callbacks
Players at the Client Side
Client has many threads interacting with each other and their Servers. In this Single
client is controlled by the server.
DebitCredit2TClient this provide the main method for the client program. It creates
an instance of the DebitCredit2Tclient by calling the class constructor. The class
constructors does the following things
- Create the user interface.
- Creates a BankDB object and connect to the database.
- Creates the new thread ClientContolThread(for the Coordinator Server to send the
callbacks).It starts the ClientControlThread at the higher priority(Callback Thread).
- Creates a DebitCreditThread at the regular priority. This thread used BankDB Object to
invokes innovation on the database(Database Thread).
ClientDebitCreditThread extends the JAVA Thread class. It implements the run method
which implements continuos loop. It generates two random number TellerID and BranchID and
invokes the method on the BankDB object which invokes JDBC invocations on the database.
ClientControlThread extend JAVA thread Class. It is the thread which register the
Client(DebitCredit2Tclient) with the Server(Coordinator). It creates the instance of the
ClientControlImpl which implements function Stop( ) and Start( ) defined at the Client
side IDL. It export the object(by Register method of the Server) to the Coordinator and
listen to the Server for the Callbacks.
ClientControlImpl this is the class which implements the Client IDL methods stop( )
and Start( ).
It implements the IDL
module Client
{ interface ClientControl
{ // start and stop operations for controlling client counting
boolean start();
string stop();
};
};
Debit-Credit 2 Tier Architecture

In this we use two Protocol CORBA and JDBC .In this 2- Tier Application we use CORBA to
Organize the benchmark.MultiConsole Applet controls the Coordinator via CORBA. Client uses
CORBA to register for the callbacks. Coordinator uses CORBA to controls the races.
Interaction Diagram for the 2-Tier Debit and Credit Scenario
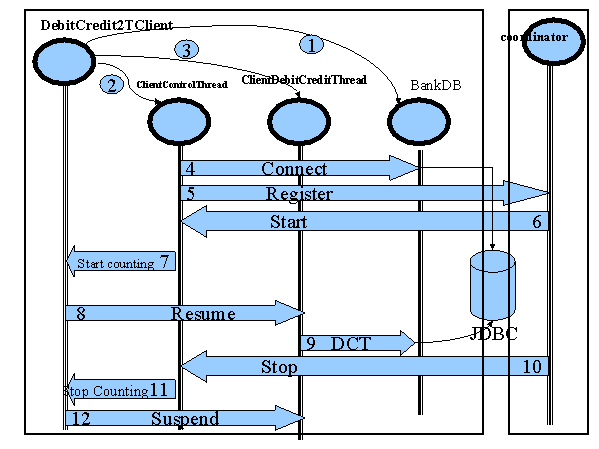
Steps of the Interaction Diagram
Steps
- Create the JDBC Bank Object
- Create the ClientControlThread for the callbacks
- Create the ClientDebitCreditThread for JDBC transactions
- Connect to the database the BankDB object using ClientControlThread
- Register for the callback with the Server
- The Coordinator tells every client to start
- Pass the Command to the main thread(DebitCredit2Tclient) to invoke startcounting Method
- Activate the ClientDebitCreditThread object
- Invoke the JDBC transactions on the BankDB using ClientDebitCreditThread
- Coordinator tells every Client to Stop
- Pass the command to the main thread(DebitCredit2Tclient)
- Suspend the ClientDebitCreditThread(JDBC transaction thread)
13.0 Summary
Comparison of Three Tier Vs Two Tier using Java ,CORBA and JDBC
|
Comparison
Field |
2-Tier
|
3-Tier |
1 |
Applet Downloading |
Slow |
Fast |
2 |
Scalability |
Very Poor (Fat Client) |
Very Good(Thin Client) |
3 |
Ease Of Deployment |
Very good |
Some What Difficult |
4 |
Separation of Concern |
Bad |
Very Good |
5 |
Ease of Development |
High |
Getting Better |
6 |
Load Balancing |
Poor |
Very Good |
7 |
System Administration |
Complex (more Logic on
Client to Manage) |
Less Complex (Application
can be Manage at Server) |
8 |
Performance |
Low |
High |
9 |
Encapsulation of Data |
Low (most of the Code is at
Client Side, Data is Exposed) |
High (Method is Exposed) |
10 |
Application Reuse |
Poor |
Excellent |
In General you see Three Tier is better than the 2-tier in many ways, 3-tier is faster
than 2-tier with the exception of JDBC, Load Balancing can be done very easily, Easy to
integrate, More secure than two tier
etc .
Appendix A
Installing and Running the Debit and Credit 2-Tier Application
Steps
- Copy the file structure from the Disk on to the drive. Let say
D:\Jdbcbank\
- Set the Classpath for the Java and the ORBIX to D:\Jdbcbank\java_output\;
- Go to the Control Panel and open ODBC by double clicking it.
- Click the User DSN tab and press Add.
- Select the ODBC driver MS Access and press Finish.
- Write the "TestDB" to the field as Data Source.
- Press Create(It will create a blank database ), give it the name bank.mdb.
- Go to the directory D:\Jdbcbank\database and compile all the classes
Prompt >javac *.java
Create database table and load the tables.
Prompt >Java CreateBank 1 TestDB
Stat the Orbix java Demon.
Compile all the classes in the MultiCoordinator directory by Prompt >javac *.java. Register the
coordinator Server in the Implementation Repository by
Prompt >puit Coordinator
MultiCoordinator.CoordinatorServer
Start the Server.
Prompt >java
MultiCoordinator.CoordinatorServer
Start the MultiConsole applet
Prompt> appletviewer MultiConsole.html
Compile all the classes in the Client directory.
Start the Client by
Prompt>java Client.DebitCredit2Tclient 1
TestDB host(what ever the host is)
Start the another Client
Prompt>java Client.DebitCredit2Tclient 2
TestDB host(what ever the host is)
Appendix B
References
Brain K. Cottman "OPENjdbc: Pure CORBA JDBC Data Access"
I-Kinetics Inc. www.i-kinetics.com,
1997
http://www.i-kinetics.com/wp/ojmgmt/ojmgmt.html
Orfali, R., D. Harkey. Client/Server Programming with JAVA and CORBA,
John Wiley and Sons, New York, NY,1996.
Siegel, J.(ed.).CORBA Fundamentals and Programming,
John Wiley and Sons, New York, NY,1996.
JavaSoft (Sun Microsystems Inc.) " JDBC - Connecting Java and Databases",
JavaSoft 1996.
Morrison, M.. Java Unleashed
Sams Net Publishing 1997
Visual Café(SYMANTEC) for Java, User's Guide
Symantec Corporation 1997
Higgs Mike "CORBA ,JAVA,JDBC ,UDAS: Universal Data Access"
I-Kinetics Inc. www.i-kinetics.com,
1997
http://www.i-kinetics.com/wp/udas/EFC_Universal_Data_Access.html
Taylor,Art . JDBC Developer's Resource
Database Programming On the Internet
INFORMIX Press CA USA ,1997
Appendix C
Source Code
The Source Code has three directory
- Jdbcbank/Database(this is to Create the Bank Database)
- Jdbcbank/Java_output/Client (this contains the Client Code in the Package Client)
- Jdbcbank/Java_output/MultiCoordinator(this contains the Server Code in the package
MultiCoordinator)
- It contains Coordinator.idl .This contains the client(as client implements callbacks)
and Server IDL.
|